Chapters
-
Course Code
Subscribe to download the code!Compatible PHP versions: >=5.5.9
-
This Video
Subscribe to download the video!
-
Course Script
Subscribe to download the script!
Field Types and Options
Scroll down to the script below, click on any sentence (including terminal blocks) to jump to that spot in the video!
Keep on Learning!
If you liked what you've learned so far, dive in! Subscribe to get access to this tutorial plus video, code and script downloads.
So far, we set the property name of the form fields and Symfony tries to guess
the best field type to render. Since isPublished
is a boolean
in the database,
it guessed a checkbox field:
Show Lines
|
// ... lines 1 - 11 |
class Genus | |
{ | |
Show Lines
|
// ... lines 14 - 41 |
/** | |
* @ORM\Column(type="boolean") | |
*/ | |
private $isPublished = true; | |
Show Lines
|
// ... lines 46 - 137 |
} |
But obviously, that's not always going to work.
Google for "Symfony form field types", and find a document called Form Types Reference.
These are all the different field types we can choose from. It's got stuff you'd
expect - like text
and textarea
, some HTML5 fields - like email
and integer
and
some more unusual types, like date
, which helps render date fields, either
as three drop-downs or a single text field.
Right now, isPublished
is a checkbox. But instead, I'd rather have a select drop-down
with "yes" and "no" options. But... you won't see a "select" type in this list. Instead,
it's called ChoiceType.
Using ChoiceType
ChoiceType
is a little special: it can render a drop-down, radio buttons or checkboxes
based on what options you pass to it. One of the options is choices
, which, if
you look down at the example, you can see controls the actual items in the drop-down.
Let's use this! In GenusFormType
, the optional second argument to the add
function
is the field type you want to use. Set it to ChoiceType::class
:
Show Lines
|
// ... lines 1 - 5 |
use Symfony\Component\Form\Extension\Core\Type\ChoiceType; | |
Show Lines
|
// ... lines 7 - 9 |
class GenusFormType extends AbstractType | |
{ | |
public function buildForm(FormBuilderInterface $builder, array $options) | |
{ | |
$builder | |
Show Lines
|
// ... lines 15 - 18 |
->add('isPublished', ChoiceType::class, [ | |
Show Lines
|
// ... lines 20 - 23 |
]) | |
Show Lines
|
// ... line 25 |
; | |
} | |
Show Lines
|
// ... lines 28 - 34 |
} |
The third argument is an array of options to configure that field. What can you pass here? Well, each field type has different options... though there are a lot of options shared by all types, like the ability to customize the label.
Either way, the reference section will tell you what you can use. Pass in the choices
option and set that to an array. Add Yes
mapped to true
and No
mapped to false
.
The keys - Yes
and No
will be the text in the drop down:
Show Lines
|
// ... lines 1 - 9 |
class GenusFormType extends AbstractType | |
{ | |
public function buildForm(FormBuilderInterface $builder, array $options) | |
{ | |
$builder | |
Show Lines
|
// ... lines 15 - 18 |
->add('isPublished', ChoiceType::class, [ | |
'choices' => [ | |
'Yes' => true, | |
'No' => false, | |
] | |
]) | |
Show Lines
|
// ... line 25 |
; | |
} | |
Show Lines
|
// ... lines 28 - 34 |
} |
The values - true
and false
will be the value that's passed to setIsPublished()
if
that option is chosen.
Try it out! Head back to the form and refresh! Perfect.
The EntityType
Let's keep going with this. This subFamily
field is also a drop-down. So that's
probably being guessed as a ChoiceType
, right? Almost. Check out the EntityType.
This is just like the ChoiceType
, except it's really good at fetching the options
by querying an entity.
In this case, it's automatically querying for the SubFamily
entity: it guessed
which type to use and auto-configured it. And yeah, I know - these sub-families
are totally silly. They're actually just the last names of people - coming from the
Faker library - I was being lazy.
Form Field Options
But I do have one problem with this EntityType
field: it auto-selects the first
option. That's lame - I'd rather have an option on top that says "Choose a Sub-Family".
Check out the options for EntityType
: there's one called placeholder
. Click to
read more about that. Yes! This is exactly what we want!
Open the form class back up. We know that Symfony is guessing the EntityType
for
the subFamily
field. We could now manually pass EntityType::class
as the second
argument. But don't! Pass null
instead. Now, add a placeholder
option set
to Choose a Sub-Family
:
Show Lines
|
// ... lines 1 - 10 |
class GenusFormType extends AbstractType | |
{ | |
public function buildForm(FormBuilderInterface $builder, array $options) | |
{ | |
$builder | |
Show Lines
|
// ... line 16 |
->add('subFamily', null, [ | |
'placeholder' => 'Choose a Sub Family' | |
]) | |
Show Lines
|
// ... lines 20 - 28 |
; | |
} | |
Show Lines
|
// ... lines 31 - 37 |
} |
But wait, why did I pass null
as the second argument? Well first, because I can!
I can be lazy here: if I pass null
, Symfony will guess the EntityType
. That's
cool.
Second, when you use the EntityType
, there's a required option called class
.
Let me show you an example: 'class' => 'AppBundle:User'
. You have to tell
it which entity to query from. But if you let Symfony guess the field type for
you, then it will also guess any options it can, including this one. So by being
lazy and passing null
, it will continue to guess the field type and a few other
options for me, like class
.
Anyways, go back, refresh, and there it is. Here are the key takeaways. First, you
have a giant dictionary of built-in form field types. And second: you configure each
field by passing a third argument to the add()
function.
Now, how could we further control the query that's made for the SubFamily
options?
What if we need them to be listed alphabetically?
42 Comments
Hey Michael K.
Symfony by itself can only take care of your back-end, if you want dynamic things going on when you click on checkboxes or filling a text box, you need to add some javascript to your page
Have a nice day :)


Ok, I got it! :-)
Thanks a lot and wish u a great day too!
I forgot to point you to this part of the documentation, I'm sure you will find it helpful :)
https://symfony.com/doc/cur...
Cheers!


Thanks!!
Have a nice day!
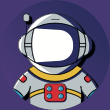
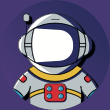
Hi there!
Let me tell you that I'm really having fun with your courses, laughing all the time. You have a gift Ryan. I'm feeling more dangerous day by day :)
Well, I know you have the easy solution to this. I have a User Entity which has a Team Entity (manyToOne relationship). The logged user can add another one just to his/her team. I was wondering if I could set a hidden field to automatically select the team where the logged user belongs to. The second option is to have a visible Choice Type, but with just one Team by default.
I've already tried with dependency injection (ha-ha-ha) using TokenStorageInterface in the constructor of the FormType class, and a HiddenType field:
public function buildForm(FormBuilderInterface $builder, array $options)
{
$user = $this->tokenStorage->getToken()->getUser();
$builder
->add('email')
->add('name')
->add('lastName')
->add('team', HiddenType::class, [
'data' => $user->getTeam()
])
;
}
But it gets the name of the team only, not the entity object. I read about DataTransformers, and another not so clean solutions.
What do you recommend me to do?
Thanks in advance and congratulations for your great work.
Yo Hugo!
You've caught onto my favorite thing to say - getting "dangerous" :). I'm really glad to hear it!
And yes, I (hopefully) do have an easy solution for you :). To summarize:
1) You have a form that modifies the User object
2) You would like the team field to automatically be set to the team of the currently-logged in User.
To do this, I want you to actually remove the team field entirely from your form. And instead, just do a little bit of work in your controller. Here's some "faux" code:
public function addUserToTeamAction()
{
$newUser = new User();
$newUser = $newUser->setTeam($this->getUser()->getTeam());
$form = $this->createForm(NewUseTeamForm::class, $newUser);
// normal form processing
}
By doing this, when you submit the form, a new User() object is created, we set its team immediately, and then the form data is added to it. By the time we persist+flush, the $newUser
variable will have the team set AND any data from the form. Unless I'm misunderstanding something, this should be your solution.
Let me know if I understood correctly! And cheers!
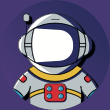
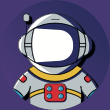
Thanks Ryan!
I did what you said, makes perfect sense. Now I have this:
public function newAction(Request $request)
{
$user = new User();
$user->setTeam($this->getUser()->getTeam());
$form = $this->createForm(UserFormType::class, $user);
$form->handleRequest($request);
if ($form->isSubmitted() && $form->isValid()) {
$user = $form->getData();
$em = $this->getDoctrine()->getManager();
$em->persist($user);
$em->flush();
$this->addFlash('success', 'The user has been created');
return $this->redirectToRoute('admin_users_list');
}
return $this->render('admin/users/new.html.twig', [
'userForm' => $form->createView()
]);
{# admin/users/new.html.twig #}
{#...#}
{{ form_start(userForm) }}
{{ form_widget(userForm) }}
<br><button type="submit" class="btn btn-primary">{% trans %}Save{% endtrans %}</button>
{{ form_end(userForm) }}
But now I get this error:
An exception has been thrown during the rendering of a template ("Notice: Array to string conversion") in form_div_layout.html.twig at line 13.
I couldn't get it to work, what could be the problem? I saw that the form has no Team, but I guess it's fine because like you said, the User object is created when I submit the form.
Hey Hugo!
The setup looks *great* - seriously, I can't see any issues... which is kind of a problem since you have this error! :). It looks like something weird is happening when rendering one of your input fields (line 13 in that file is responsible for that (https://github.com/symfony/.... Can you show me your form class? And also, a screenshot of the full exception page when you get that error? It's going to be something subtle...
Cheers!
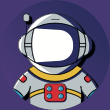
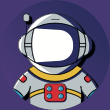
Sure!
Here it is the form class
class UserFormType extends AbstractType
{
public function buildForm(FormBuilderInterface $builder, array $options)
{
$builder
->add('email')
->add('name')
->add('lastName')
->add('interiorNumber')
->add('phoneNumber')
->add('mobileNumber')
->add('plainPassword')
->add('roles');
}
public function configureOptions(OptionsResolver $resolver)
{
$resolver->setDefaults([
'data_class' => 'AppBundle\Entity\User'
]);
}
public function getName()
{
return 'app_bundle_user_form_type';
}
}
<a target="_blank" href="https://dl.dropboxusercontent.com/u/3747475/Captura de pantalla 2016-10-31 a la(s) 23.57.55.png">Here is the ContextErrorException 1/2</a>
<a target="_blank" href="https://dl.dropboxusercontent.com/u/3747475/Captura de pantalla 2016-10-31 a la(s) 23.57.31.png">Here is the Twig_Error_Runtime 2/2</a>
Please tell me if you need something more about the exception page.
Thanks!
Hey Hugo!
Hmm, that form is perfectly boring :). But I think I might see the problem: it's the "roles" field. Is that an array (e.g. json_array) type in your User class like how we setup in this tutorial? In that case, Symfony is likely guessing that this is a text field (that's it's default) and then choking when it tries to get that array value and print it in the value=""
attribute. If I'm right - and you look closely - you might see the word "role" somewhere in the stacktrace of those errors (it might be a bit buried!).
Instead of using a text field, you'll probably want something like a ChoiceType
with options 'multiple' => true
and 'expand' => true
. You would set the choices
option to an array of whatever roles that you want the user to be able to select :). Or, if you have some more complex scenario, let me know!
Cheers!
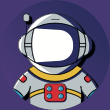
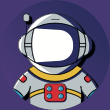
It's a boring form indeed! is because I was just getting started :P, but soon they'll be fun hopefully. I see, I thought it had something to do with the Team entity.
I was thinking about creating a select element instead, but those tags you suggested are way better, much easier and totally match with its goal.
I love this Simfony Forms.
Thanks a lot Ryan, you're the best!


Hello, I'm trying to use ChoiceType to create 'choices' but the choices are strings, not a boolean:
I'm not sure what the Option should be for each string, when set up with true, I get a 1 in the database as expected.
Any suggestion for the Option to get the strings in the 'choices' list populated?
->add('printProcess', ChoiceType::class, array(
'choices' => array(
'Flexography' => true,
'Offset Lithography' => true,
'Rotogravure' => true,
)
))


Well this seems to work, key and value the same:
->add('printProcess', ChoiceType::class, array(
'choices' => array(
'Flexography' => 'Flexograpy',
'Offset Lithography' => 'Offset Lithography',
'Rotogravure' => 'Rotogravure',
)
))
Hey Rob,
Btw, in Symfony 3 the key of "choices" option is a label you'll see in your form, but its value is a value you'll get in DB. Some devs lowercase values and replace spaces with underscores like:
->add('printProcess', ChoiceType::class, array(
'choices' => array(
'Flexography' => 'flexograpy',
'Offset Lithography' => 'offset_lithography',
'Rotogravure' => 'rotogravure',
)
))
But of course it's up to you ;)
Cheers!


Good advice on the lowercase and underscores, thank! I did that and then used:
<td>{{ profile.printProcess|title|replace({'_': ' '}) }}</td>
to get back the original string. Perfect!


Thanks Victor!
Wow, cool! Btw, very often devs use translator instead. The reason is that forms labels are always translated out-of-the-box. So you can translate them in app/Resources/translations/messages.yml:
offset_lithography: Offset Lithography
flexograpy: Flexography
P.S. Ensure the translator is enabled in your config file.
Cheers!


Did not know about translator, thanks. After setting up the messages.yml and enabling translator in the config file, what would the twig tag look like? I'm trying like: {% trans %} {{profile.printProcess}} {% endtrans%} or {% trans %} %profile.printProcess% {% endtrans%} but that's not it... Can we get the message translated simply with a twig tag?
Yes, "trans" tag should work fine, but I prefer the "trans" filter: {{ profile.printProcess|trans }} - it's simpler. After setting up the messages.yml and enabling the translator in the config file you have to manually clear the cache, even in dev environment - this is one of a few places where you have to clear the cache first. Probably you know, but here's a link to the Symfony docs just in case. Let me know if it still doesn't work for you.
Cheers!


Hmm... not getting the translation with {{ profile.printProcess|trans }}
Could it be something with my messages.yml file?
app/Resources/translations/messages.yml
messages:
offset_lithography: Offset Lithography
flexography: Flexography
I do have the translator enabled by removing the # and have cleared the cache.
Also tried without messages: in Line 1
Not that big of a deal right now but if you have a suggestion that might help, thank you in advance Victor.
Oh, man, my fault! I forgot to mention about language of this messages file, so the right file should be: "app/Resources/translations/messages.en.yml" if default locale for your application is set to "en". So even if you don't have multi-language website - you still have to point the language in your file's name.
UPD: And yes, you just need to specify keys there, don't need to add "messages:" level, e.g. in your messages.en.yml write:
offset_lithography: Offset Lithography
flexography: Flexography
P.S. Probably you still have to clear the cache (both prod and dev) after creating this messages.en.yml file. )
Cheers!


Thanks Victor. It does work on my show page but not on my list page. These pages are set up just like the lessons; the list.html.twig page goes through the for loop and the show.html.twig page finds one by 'name'
Hey Rob,
Do you mean that "offset_lithography" message key is not translated on your list page? It's weird if it works on show page but not on the list page. Is the code the same? If it is, probably you have to clear the cache, I don't have other ideas. :/
Cheers!
Hey @thisismattness!
Yes! In fact, that's exactly how the EntityType field *should* work out-of-the-box... but a lot depends on your setup :). Basically, when you have an EntityType with multiple=true, when you submit, Symfony process the incoming, checked ids, turn those into your entity objects, and then set that array of entities onto the property on your main entity (whatever field name you're using in your form for the EntityType). And also, when the form *renders*, it does the opposite: it uses your query to get all of the entity objects for the choices, then gets the array of entity objects from that same property and compares them. For example, suppose I have a Category entity with a products property. I then add a products field to my form, which is an EntityType. When the form renders, it uses my custom query on that field to load all (for example) 25 products in my system. It then looks at the products property on Category, which holds (for example) 10 of those products. So now, it knows to render 25 checkboxes, with 10 of them checked.
That's a long way of saying that something might not be setup correctly if you're not getting your boxes auto-checked. Let me know if this explanation helped! And feel free to come back with some of your code - it'll help show what's going on :).
Cheers!
Hey Matt!
Ah, it *does* help :). So, what you're doing makes great sense, but is a little non-traditional. The EntityType is meant to be attached to a property whose value is an *object*... basically a ManyToOne property. But, your name field is a string. Based on what I'm seeing, if you submit right now and dump your final value, the name property will be an array of Chapter objects (not a string). (I'm also a little confused about how you have a "name" field, but the user is selecting multiple checkboxes - so I could be misunderstanding something!)
But, you totally *can* do this, you just need to do a little bit more work :). Basically, you should use the ChoiceType field with a custom data transformer that's able to convert to and from the "text" of the name field and the Chapter entity object (or objects, if you really do intend for this to be multiple checkboxes). You'll approximately be following this tutorial: http://symfony.com/doc/curr...
Let me know if this helps... or confuses further :).
Cheers!


Hi,
I want to know how to handle FileType with an entity. I've got products and productImages. But I can't seem to find out why I cannot save this to the database. It does upload the file, but I get an error with saving.
http://stackoverflow.com/qu...
here's the stackoverflow question I asked for it.
Hey Dennis!
That's a nice question, there is an extension that can help you with the task "Gedmo Uploadable":
https://github.com/Atlantic...
You will have to create a File Entity as you did it with *ProductImage* but using this extension now, then you will have to add the relationship to your *Product* class.
This extension is well documented so I hope you won't have so much troubles ;)
Have a nice day!


Hi Diego,
Thanks for your answer, but I've already solved it. (thanks to stackoverflow)
I did the same as Ryan in the tutorial, but Ryan only persists the $product, and I had to also persist $productImages.
Thanks tho for your answer :)
You have a nice day too there at KNP University.
I'm glad you fixed your problem :)
FYI: If you don't want to persist "ProductImage" entity explicitly, you can configure the "cascade" option to "on persist" in your relationship
you can find more information about it here:http://docs.doctrine-projec...
Or watch our tutorial about doctrine collections (which I think is great): https://knpuniversity.com/s...
Cheers!


Hello, Ryan! Thanx a lot for your voice in my head )) I don't understand if I has some ORM field that could be empty, am I have to make it "nullable"? I tried to pass an empty string as default value (private $some = '') but always get an error that a column cannot be null... I feel that nullable (if it's work) is not a good practice, please give an advice
Hey Dmitry V.
Is totally ok to set a not null constraint to a field, and that constraint can be set for two different layers, DB and forms. If you don't like it, you would have to set a default value that is not considered empty
Cheers!
Hey Matt!
Coding for a few weeks now! Wow! Congrats! And don't sweat too much about this form stuff - forms is the hardest part of Symfony (it's also powerful and rewarding... but can easily be frustrating, even for really seasoned devs!).
Ok, I think I definitely understand your setup - the jsfiddle was great. Afterall, backend code ultimately exists just to power some UI :). Here's why I think this is confusing you: the purpose of your form is not to update an entity. What I mean is, most of the time, we create a form so that we can update or create some entity. For example, if you wanted to create a form to allow the user to create a Chapter, that would be pretty easy for you: your form would be bound to the Chapter class, you would have a field for each property in your entity, and the types of those fields would naturally be simple.
But in your case, your form isn't doing that: the purpose of you form is simply to create a checkbox of chapters. And when you submit, you just want to fetch what chapters were checked, so you can redirect the user. So, here's the key: I want you to create a form that is not bound to an object. If you build it in your controller, it should look something like this:
// the form is not bound to an object. Instead, we just pass it an associative array of
// "starting" data, that should be used to pre-fill the form
$defaultData = [
// I don't know if this is right - the point is, you should set a "chapters" key to the
// array of Chapter objects that should be pre-filled
'chapters' => $quotes->getChapters()
];
$myForm = $this->createFormBuilder($defaultData)
->add('chapters', EntityType::class, [
// this should be the entity class that should be used for the checkboxes
// I'm assuming you want a checkbox for each Chapter
// notice there is no logic here to say which chapters should be pre-checked, that happens above
'class' => 'AppBundle\Entity\Chapter',
// I'm assuming the Chapter has a name property - just kinda made that up :)
'choice_label' => 'name',
'multiple' => true,
'expanded' => true
]
)
->getForm();
$form->handleRequest($request);
if ($form->isValid()) {
$formData = $myForm->getData();
// this will be an array of Chapter objects, the ones the user selected
/** @var Chapter[] $chapters */
$chapters = $formData['chapters'];
// loop over $chapters to create your ?chapter=chapter_foo&chapter_bar query string, and then redirect to a route with this appended to it
$queryString = '...';
$url = $this->generateUrl('result_route_name').'?'.$queryString;
return $this->redirect($url);
}
Let me know if this setup makes sense, and if I've finally hit on the problem! Focus on this idea: the EntityType's job is simply to create a bunch of checkboxes (in this case) for all the records for some entity (e.g. Chapter). You can of course use the query_builder option to filter that list from all Chapters to some Chapters. But, the options you pass to EntityType have nothing to do with which checkboxes should be checked by default: that's entirely up to what data you pass into your form. And in this case, we're not binding our form to any entity, we're just passing it an associative array, and it's giving us an associative array after (i.e. $form->getData() is an associative array). We're not binding it to an entity because it's not really necessary: it's a really cool "nice-to-have" when you eventually want to update an entity and persist it. But in this case, all we need is that our form gives us an array of the Chapter objects that were checked. Then, we're happy :).
Cheers!
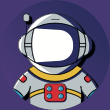
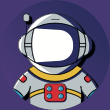
How to control input in the fields ? In other words, what if I want to make sure the user doesn't click "add" with a field being filled with only spaces for example ? Or with words > 10 characters, or any other checking I want to add. How to do that? Will it be explained later on? ^_^
Hey Islam Elshobokshy!
Of course! Validation :). We do talk about it a little bit later: https://knpuniversity.com/s.... Specifically, you should look at the Length constraint (http://symfony.com/doc/curr... and also Callback (http://symfony.com/doc/curr... - that's a really handy one :).
Cheers!
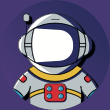
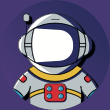
Hello,
I have a problem with the isPublished field. The HTML for options is generated as follows:
<option value="1">Yes</option>
<option value="" selected="selected">No</option>
so, "false" is parsed as an empty string and the option is marked as default. On form submit, with "No" selected, the browser (Firefox 47.0) shows the balloon "Please select an item in the list". When "Yes" is selected there's no balloon.
When I change the field settings and map "Yes" to "1" and "No" to "0", it works:
<option value="1">Yes</option>
<option value="0">No</option>
Why is that?
Hi Grzegorz!
Hmm, you're right! The behavior is not seen in Chrome, but it is seen in Firefox - this is one of the crazy things about HTML5 validation :).
In short, I think this may be a bug in Symfony - these areas can be quite complex: https://github.com/symfony/.... These types of things aren't easy to fix without breaking backwards compatibility.
So, that's the short answer :). And other than the HTML5 issue, Symfony is really doing the correct thing - it needs to somehow map our "true" and "false" to values, and 1 and an empty string are a valid way to do this (though "1" and "0" would have made more sense).
Thanks for the question about this - it was not something I had realized!
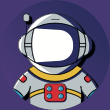
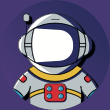
Hi Ryan,
Thanks for the link. I knew casting or mapping was broken, but would never think it's that complex.
Hey Matt!
You got it! It's important that your form field name "chapters" matches a key on your incoming/starting data. Usually, we bind a form to an object, so we would expect to have a "chapters" property on our object, which it would use to know which boxes to check. But in this case, since we're binding the form to an array, it's simply an array key. You got the "aha!" moment I was hoping you'd get :).
Cheers!
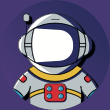
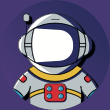
Hi,
Regarding the placeholder, in formType.
// method 1: This works.
->add('name', TextType::class, array(
'attr' => array('placeholder' => 'Please type in your name here.')
))
//Method 2: As suggested in the video, but, this did not work for me...
->add('category', null,
['placeholder' => 'Type in its category.']
)
And I get the below error when using Method 2 to create a placeholder for input field...
Is there anything I forgot to make it work?
The option "placeholder" does not exist. Defined options are: "action", "allow_extra_fields", "attr", "auto_initialize", "block_name", "by_reference", "compound", "constraints", "csrf_field_name", "csrf_message", "csrf_protection", "csrf_token_id", "csrf_token_manager", "data", "data_class", "disabled", "empty_data", "error_bubbling", "error_mapping", "extra_fields_message", "inherit_data", "invalid_message", "invalid_message_parameters", "label", "label_attr", "label_format", "mapped", "method", "post_max_size_message", "property_path", "required", "translation_domain", "trim", "upload_max_size_message", "validation_groups".
500 Internal Server Error - UndefinedOptionsException
Hey Nobuyuki!
You have a tiny error and to be honest it happens to me frequently :p
the placeholder option should be inside the "attr" option. Give a deeper look to your "method 1" and you will see it :)
You can check all the options for a TextType here: http://symfony.com/doc/curr...
Cheers!
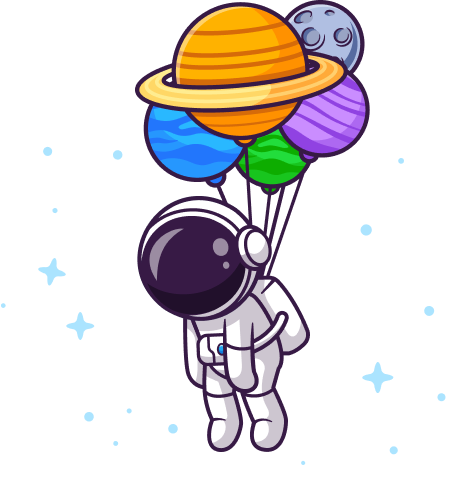
"Houston: no signs of life"
Start the conversation!
What PHP libraries does this tutorial use?
// composer.json
{
"require": {
"php": ">=5.5.9",
"symfony/symfony": "3.1.*", // v3.1.4
"doctrine/orm": "^2.5", // v2.7.2
"doctrine/doctrine-bundle": "^1.6", // 1.6.4
"doctrine/doctrine-cache-bundle": "^1.2", // 1.3.0
"symfony/swiftmailer-bundle": "^2.3", // v2.3.11
"symfony/monolog-bundle": "^2.8", // 2.11.1
"symfony/polyfill-apcu": "^1.0", // v1.2.0
"sensio/distribution-bundle": "^5.0", // v5.0.22
"sensio/framework-extra-bundle": "^3.0.2", // v3.0.16
"incenteev/composer-parameter-handler": "^2.0", // v2.1.2
"composer/package-versions-deprecated": "^1.11", // 1.11.99
"knplabs/knp-markdown-bundle": "^1.4", // 1.4.2
"doctrine/doctrine-migrations-bundle": "^1.1" // 1.1.1
},
"require-dev": {
"sensio/generator-bundle": "^3.0", // v3.0.7
"symfony/phpunit-bridge": "^3.0", // v3.1.3
"nelmio/alice": "^2.1", // 2.1.4
"doctrine/doctrine-fixtures-bundle": "^2.3" // 2.3.0
}
}
Hi there,
First, I'm a Symfony beginner, but with KnpUniversity I had learned a lot so far! :-)
I want to make a form with a checkbox and when the user check this checkbox, there should display another field, which was hidden by default. Short: display a field depending on a checkbox ;-)
I found a solution with jQuery. Is there a way to this only with Symfony?
Best regards
Michael